Lists and Arrays are a very powerful type of variable that can store big volumes of data. After storing, the data can be easily manipulated by native functions, as sorting, counting appending, etc.
The program bellow input data into the lists and then it use the data:
fruit = []
initialstock = []
sold = []
instock = []
fruit.append(input("Enter the name of the 1st fruit: "))
fruit.append(input("Enter the name of the 2nd fruit: "))
print()
initialstock.append(input("Enter the amount of kg for "+fruit[0]+": "))
initialstock.append(input("Enter the amount of kg for "+fruit[1]+": "))
print()
sold.append(input("Enter the Kg that are sold for "+fruit[0]+": "))
sold.append(input("Enter the Kg that are sold for "+fruit[1]+": "))
print()
instock.append(float(initialstock[0]) - float(sold[0]))
instock.append(float(initialstock[1]) - float(sold[1]))
print("%-30s %15s %15s" % ("Fruit", fruit[0], fruit[1]))
print("%-30s %15.2f %15.2f" % ("Initial Stock - Kg", float(initialstock[0]), float(initialstock[1])))
print("%-30s %15.2f %15.2f" % ("Sold - Kg", float(sold[0]), float(sold[1])))
print("%-30s %15.2f %15.2f" % ("In Stocl - Kg", float(instock[0]), float(instock[1])))
Copy, paste, and run this code to get the output shown below:
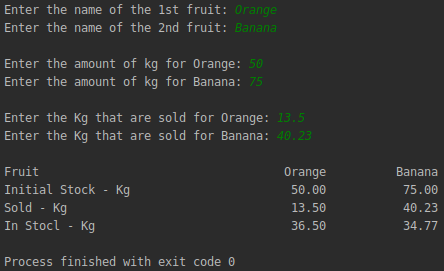